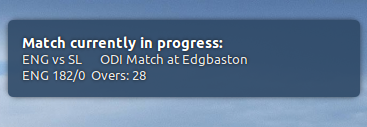
Cricket Score Notifier
- 1 minBring a regular follower of cricket, I was lazy to open espncricinfo to check the scores everytime. Then I got an Idea to write a script to notify me with present cricket match scores every 5 minutes. Without any delay I wrote the below script.
I used pynotify to create popup for score and json from espncricinfo to extract the score
Code :
Please comment your views/suggestions below.